tl;dr
- This is a fairly simple Maze challenge
- Challenge is written in rust
Challenge author: Freakston, silverf3lix
Descrption
Senpai plis find me a way.
Solution
This is a fairly simple maze challenge implemented in rust.
At the start of the main function of the challenge miz we can see there is a some sort of a initialization going on at a function named miz::bacharu::Kikai::new::h3a113b790cc2bb5c
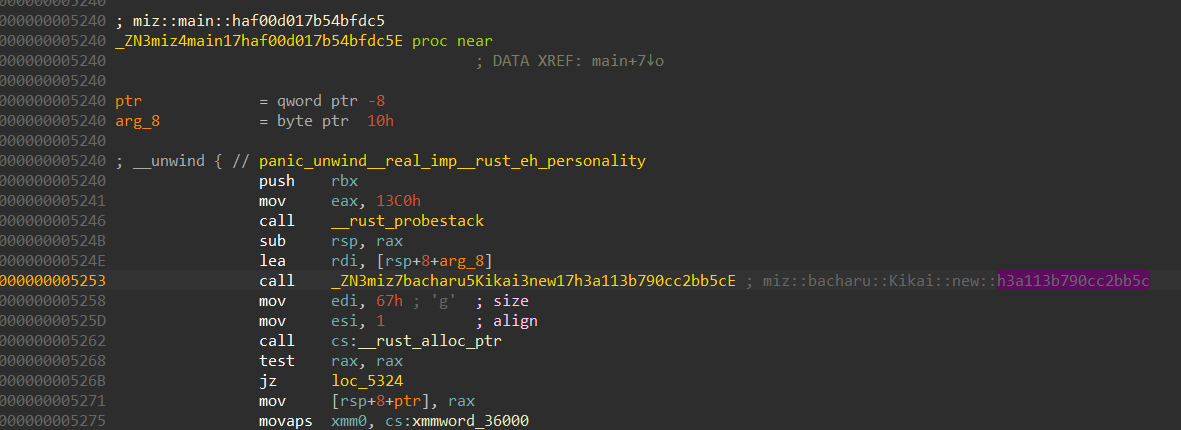
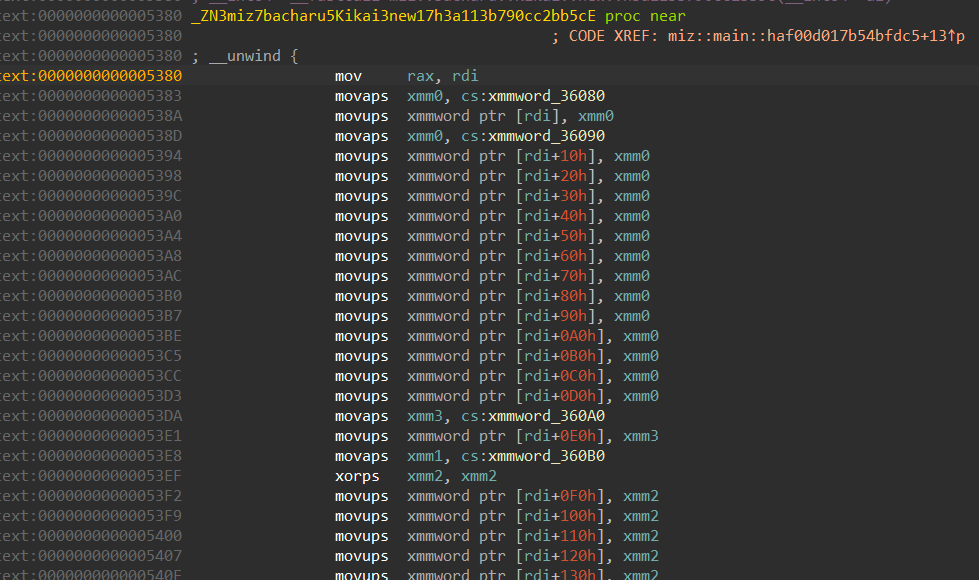
We can see from here than this function takes care of initializing the maze. We can extract the maze bytes either directly from here or during runtime, which ever is preferred.
Moving forward we can see that the function is also getting our input, and sending it over to another function miz::bacharu::Kikai::run::h14398f1fc265e61e
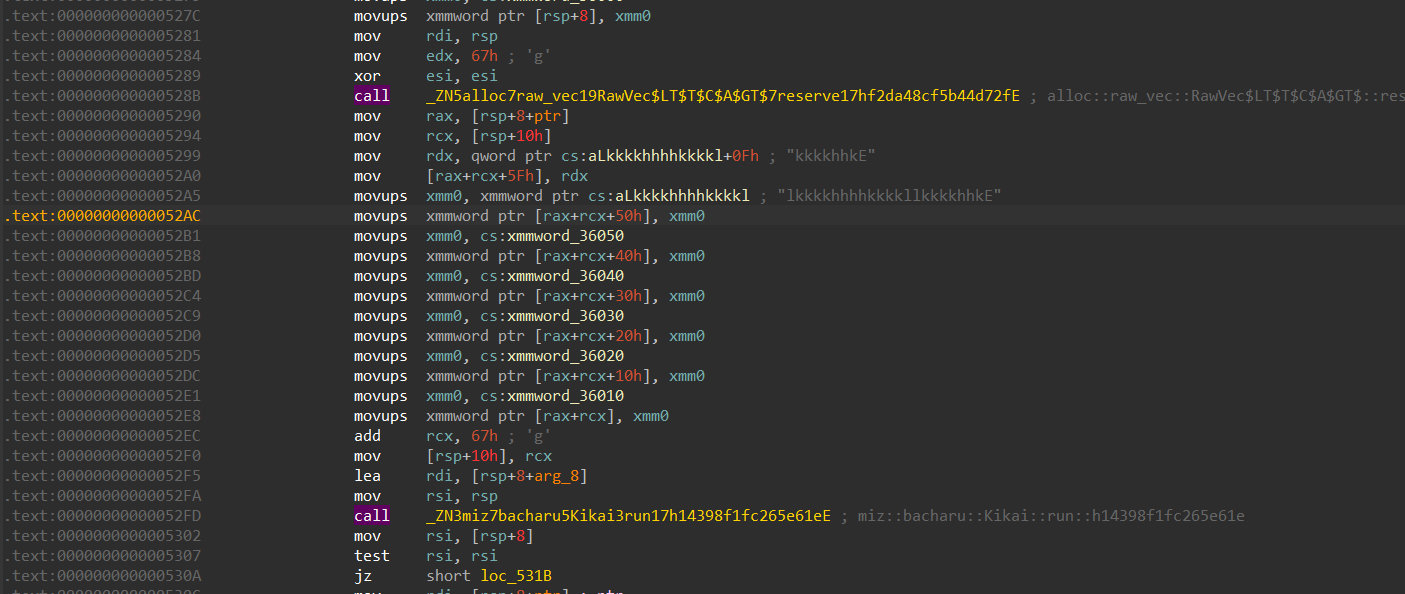
The function miz::bacharu::Kikai::run
takes care of the maze logic, the up, left, down and right.
- Case “h”
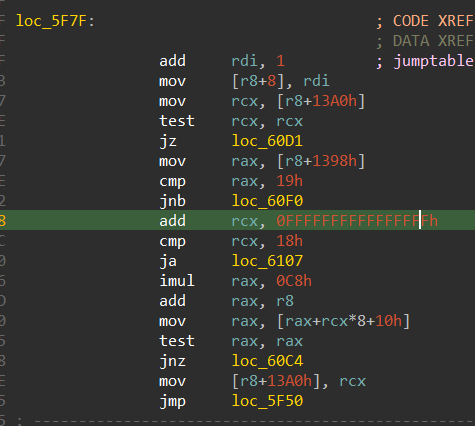
We can see that the case “h” takes care of going left, which is by subtracting 1 from the y coordinate
- Case “j”
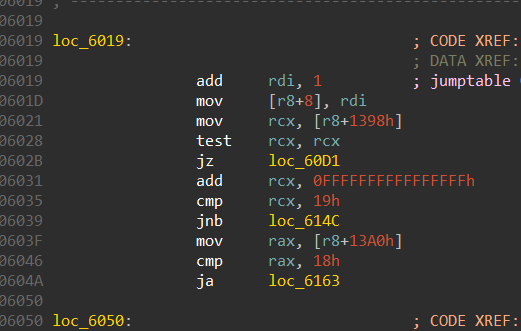
Similarly, case “j” is to go up, it does this by subtracting 1 from the x coordinate.
- Case “k”
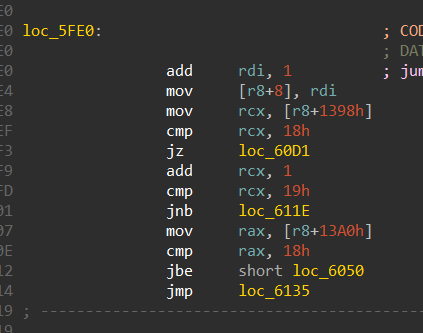
Case “k” takes care of going down the maze, it adds 1 to the x coordinate
- Case “l”
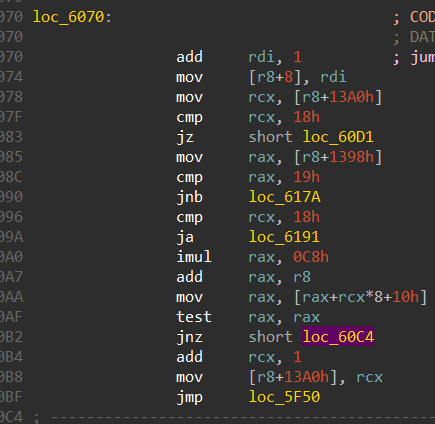
This case takes care of going right in the maze, it does so by adding 1 to the y coordinate
From this function we can also get the bounds of the maze which is 24 x 25, where there are 25 rows and 24 columns.
And the properties of the maze are,
- “0” ⇒ Path to traverse
- “1” ⇒ Walls
- “2” ⇒ Final win position
We can also see that this is the function miz::bacharu::Kikai::Furagu_o_toru::hd3e3c2fb2ccf3552
is the win function, and this is called when we traverse till we reach the number 2 in the maze.
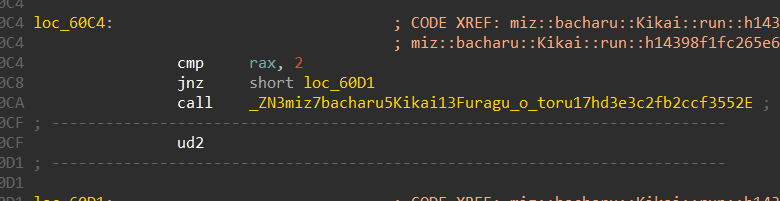
Constructing the maze should be easy since we have its bounds,
1 | miz = [[1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1] |
The start position can be retrieved while debugging and it is (0, 13). The end position is
(24, 19)
Final script
To solve this maze we can make use of the python library mazelib
Here is the script,
1 | from mazelib import Maze |
The final moves to be sent as input comes out to be,
llkkhhhhkkkkhhhhjjhhhhhhkkllkkkkkkhhkkllkklljjlllllljjhhjjllllllkklljjllkklljjllkkkkhhhhkkkkllkkkkhh
Flag: inctf{mizes_are_fun_or_get}